Manage Groups
For dynamic load management, you can group together chargestations within the same site/campus/building. Each group has
maximum_capacity
(grid capacity constraint in kilowatt)minimum_connector_capacity
(minimum power required to sustain a charging session in kilowatt)buffer
(a percentage from 0.0 to 1.0 of the maximum_capacity, set aside as a power buffer)
By setting these three parameters, eDRV's dynamic load management algorithms mitigate the risk of overloading the grid even when all chargers in the groups are being used together.
Create Groups
You can create groups for load management via POST /v1.1/load_management/groups
API or Admin Panel. Each group object contains the maximum capacity and connector level minimum capacity.
via API
const request = require('request');
const options = {
method: 'POST',
url: 'https://api.edrv.io/v1.1/load_management/groups
',
headers: {
Accept: 'application/json',
'Content-Type': 'application/json',
Authorization: 'Bearer API_KEY'
},
body: {
"name": "Park3-E1",
"description": "Airport Parking Floor 3 - East Side",
"notes": "Supports 100kW peak power demand. Recommend a 10% buffer",
"maximum_capacity": 100,
"minimum_connector_capacity": 2.5,
"buffer": 0.10,
"location": "649be7ca525c000bc98a6c05"
},
json: true
};
request(options, function(error, response, body) {
if (error) throw new Error(error);
console.log(body);
/*Save rate Id within your appication*/
const groupId = body.result._id;
});
via Admin Panel
Go to Location Details Page > Load Management Tab > Create
Add/Remove Chargestations from a Group
Use POST /v1.1/load_management/groups/{id}/chargestations
API or Admin Panel to add or remove the chargestations from a group.
Add Chargestation via API
const request = require('request');
const options = {
method: 'POST',
url: 'https://api.edrv.io/v1.1/load_management/groups/647578b4caae4a0bbcdf6d1e/chargestations',
headers: {
Accept: 'application/json',
'Content-Type': 'application/json',
Authorization: 'Bearer API_KEY'
},
body: [{
"connectors": [
{
"id": "647578b4caae4a0bbcdf6d1e",
"maximum_capacity": 15,
"minimum_capacity": 5,
"connector_id":1,
}
],
"chargestation": "647578b4caae4a0bbcdf6d1e"
}],
json: true
};
request(options, function(error, response, body) {
if (error) throw new Error(error);
console.log(body);
/*Save rate Id within your appication*/
const groupId = body.result._id;
});
Remove Chargestation via API
const request = require('request');
const options = {
method: 'DELETE',
url: 'https://api.edrv.io/v1.1/load_management/groups/chargestations/647578b4caae4a0bbcdf6d1e',
headers: {
Accept: 'application/json',
'Content-Type': 'application/json',
Authorization: 'Bearer API_KEY'
},
};
request(options, function(error, response, body) {
if (error) throw new Error(error);
console.log(body);
const groupId = body.result._id;
});
Add/Remove Chargestation via Admin Panel
To Add a Chargestation in a group
Go to Location Details Page > Load Management Tab > Click on + Add Chargestation
To Update Chargestation capacities or Remove Chargestation from the group
Go to Location Details Page > Load Management Tab > Expand the Group click on Update or Remove
Get Groups Info
Use GET /groups
or GET /groups/{id}
API's or Admin Panel to get the groups details.
via API
Get Group Details by Id
const options = {method: 'GET', headers: {
Accept: 'application/json',
Authorization: 'Bearer API_KEY'
}
}};
fetch('https://api.edrv.io/v1.1/load_management/groups/61a80bb1a43d8d4900475a3e', options)
.then(response => response.json())
.then(response => console.log(response))
.catch(err => console.error(err));
Get All Groups
const options = {method: 'GET', headers: {
Accept: 'application/json',
Authorization: 'Bearer API_KEY'
}
}};
fetch('https://api.edrv.io/v1.1/load_management/groups', options)
.then(response => response.json())
.then(response => console.log(response))
.catch(err => console.error(err));
Delete Groups
Use DELETE /groups/{id}
API's or Admin Panel to delete a groups.
via Admin Panel
Go to Location Details Page > Load Management Tab > Click on Delete
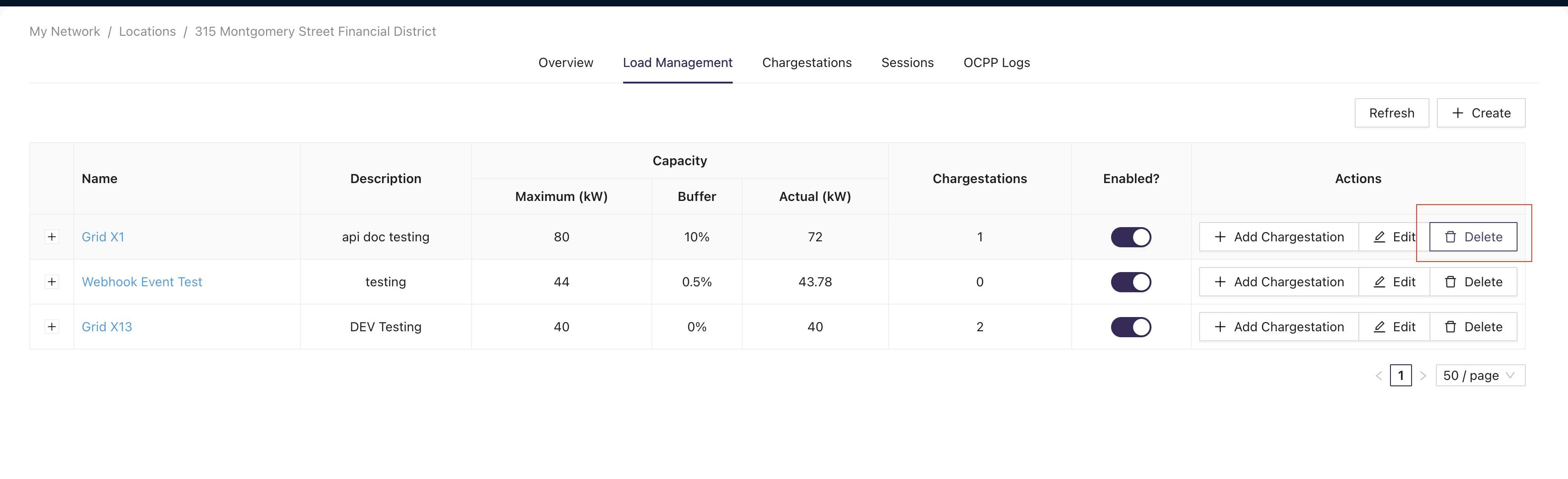
via API
const request = require('request');
const options = {
method: 'DELETE',
url: 'https://api.edrv.io/v1.1/load_management/groups/647578b4caae4a0bbcdf6d1e',
headers: {
Accept: 'application/json',
'Content-Type': 'application/json',
Authorization: 'Bearer API_KEY'
},
};
request(options, function(error, response, body) {
if (error) throw new Error(error);
console.log(body);
const groupId = body.result._id;
});
Updated about 1 year ago