Webhooks
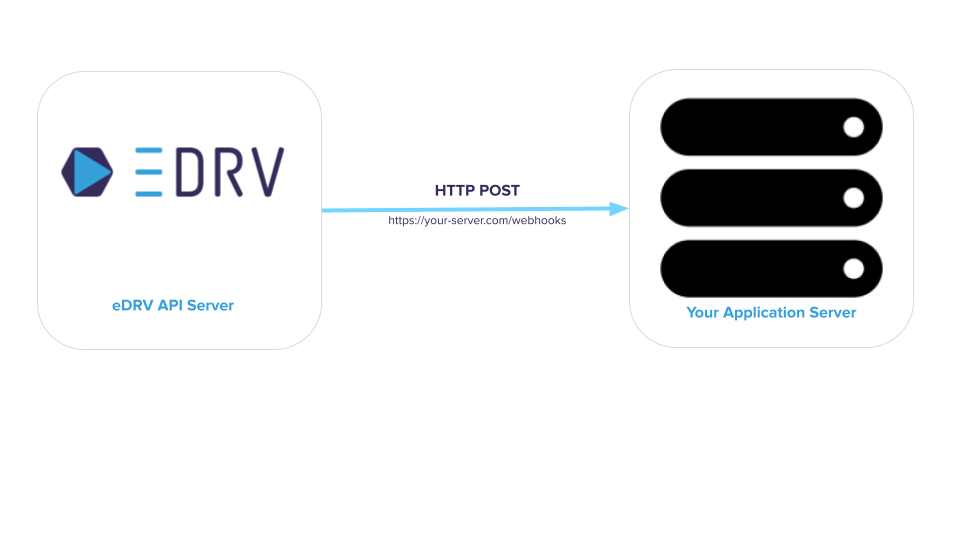
Webhook Events
Introduction
eDRV provides developer applications a near real time stream of network wide events via Webhooks. We highly recommend developers use Webhooks to listen for events instead of polling for data.
Event Types
For further information on each event type and the data fields, see For an exhaustive list of events please see Webhook Events and Data Hierarchy
Use Cases
Some of the possible use cases for Webhooks are:
- Build No-Code applications: Connect your EV charging network to thousands of other apps via Zapier and IFTTT. See Zapier Incoming Webhooks
- Integrate with Mapping providers: Report the availability of your chargestations in real time to drivers
- Onboard Users: Send a welcome set of emails helping Users who have just registered on eDRV
- Smart Charging: Build intelligent power management applications for EV charging and react in real time to upstream power congestion. See Real Time Session Power Management
- Reservation Systems: Use real time connector status events to build a queueing system for drivers in real time.
Subscribe to Webhook Events
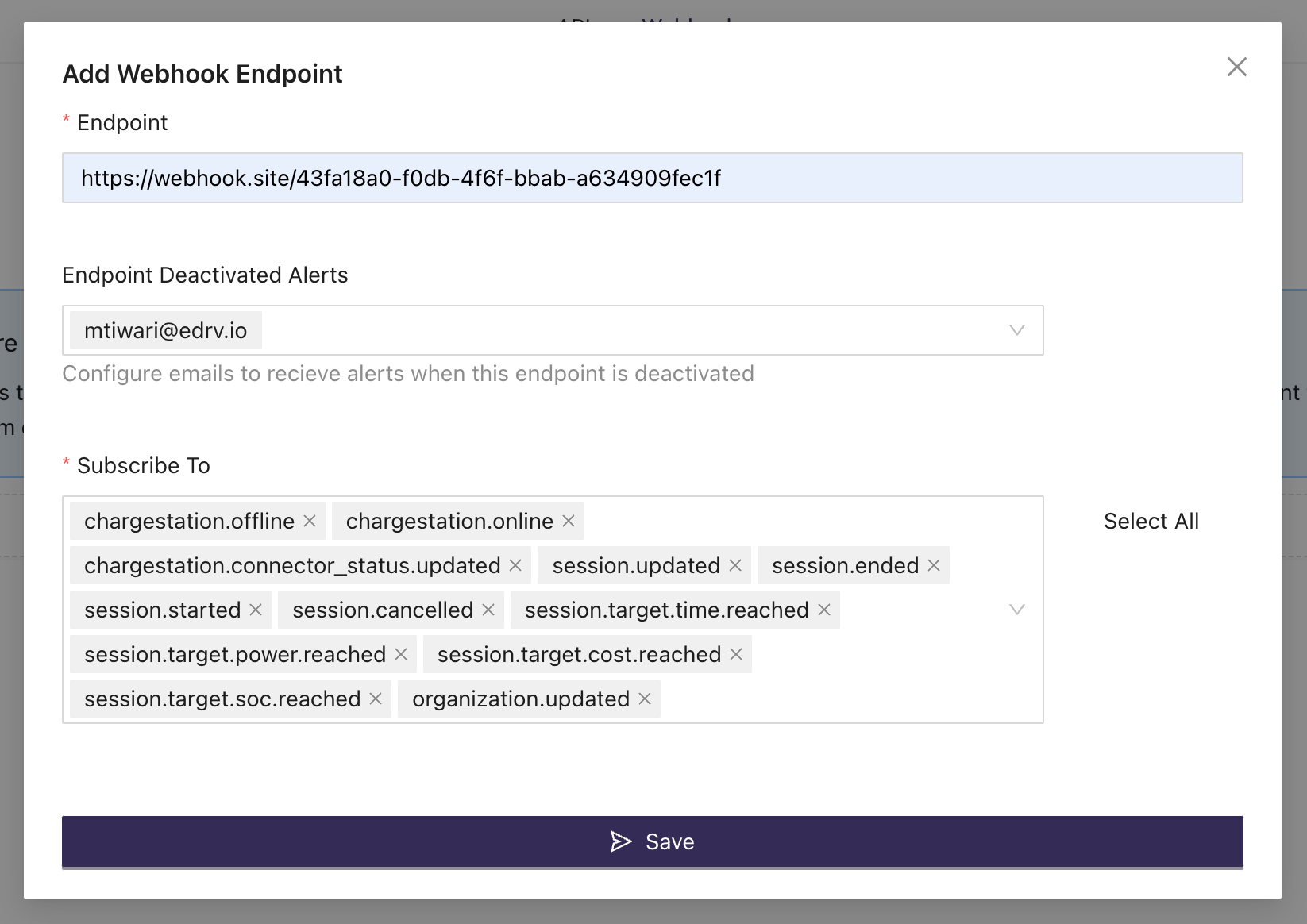
Navigate to the edrv Dashboard > Developers > Webhooks
- Input an endpoint.
- Add email to receive alerts for deactivation of this endpoint. Default set to Organization Admin
- Choose the events you want to subscribe to.
Expected Response
Your application servers must respond to each request with
200
OK status code- Empty response Body
No response or other errors
If there is continuously no response from your application or there are other errors in the POST request, eDRV will automatically disable your Webhook. An alert email will be dispatched to both the organization's email address and any custom email addresses configured with the webhook.
Processing Webhook Event Data
The Webhook Body contains the following fields that your application must handle:
_id string
Webhook Id
data string
The full data object affected by the event
object string
Core eDRV data asset type. See Data Hierarchy
type string
Event trigger type. See List Of Webhook Events for a full list.
createdAt datetime
Timestamp of this event
{
"_id": "615ab6d99ab98b057eba7e26",
"data": {
"_id": "60e7116e15f9305d44e9d4d5",
"user": "60e7116e15f9305d44e9d4d5",
"chargestation": "60e7116e15f9305d44e9d4d5",
"connector": "60e7116e15f9305d44e9d4d5",
"status": "started",
"rate": "603763d392204c0c840578be",
"cost": {
"amount": 17.5,
"currency": "eur"
},
"metrics": {
"chargingStart": "2021-07-08T14:56:47.528Z",
"chargingStop": "2021-07-08T14:55:55.000Z",
"energyPeriod": 2476,
"energyConsumed": 4406
},
"energy_report": {
"timestamp": "2021-07-08T14:55:33Z",
"current": {
"value": 31.16,
"unit": "A"
},
"power": {
"value": 7.018,
"unit": "kW"
},
"energy_meter": {
"value": 15.83,
"unit": "kWh"
}
},
"meta_data": {
"session_source": "Android_App"
},
"createdAt": "2021-07-21T12:50:36.660Z",
"updatedAt": "2021-07-21T12:50:36.660Z"
},
"object": "session",
"type": "session.updated",
"createdAt": "2021-10-04T08:34:10.863Z"
}
Demo Webhook Server
As Webhook server in Node.js with some examples on how you can process the data delivered by eDRV within your application.
var express = require('express');
var app = express();
const port = 3000;
app.use(express.json());
app.listen(port, () => {
console.log(`Listening on port: ${port}`)
});
app.route('/').get(function (req, res) {
res.send('Sample Webhook Handler');
})
app.route('/webhook').post(function (req, res) {
//Immideately respond to eDRV servers
res.send();
//Process an event
let [data,object,type] = req.body;
switch (object) {
case 'session':
if (type === "session.started") {
console.log(`New charging session started at: ${data.createdAt}`);
}
if (type === "session.updated") {
console.log(`New update for Session ${data._id} at: ${data.updatedAt}`);
//Extract updated session metrics, cost etc here
let [cost,metrics,energy_report] = data;
//Update your client apps, drivers and dashboards with this new info
}
//Process other session events here
break;
case 'users':
//Process user events here
break;
case 'chargestations':
//Process chargestation events here
break;
case 'connectors':
//Process connector events here
break;
default:
console.error(`Sorry, unrecognized event type: ${type}`);
}
});
Quick View
If you want to quickly test Webhook data received you may consider a disposable Webhook endpoint from a service like Webhook.site.
Remember to delete your disposable webhook from the eDRV dashboard after you have tested it.
Updated over 1 year ago